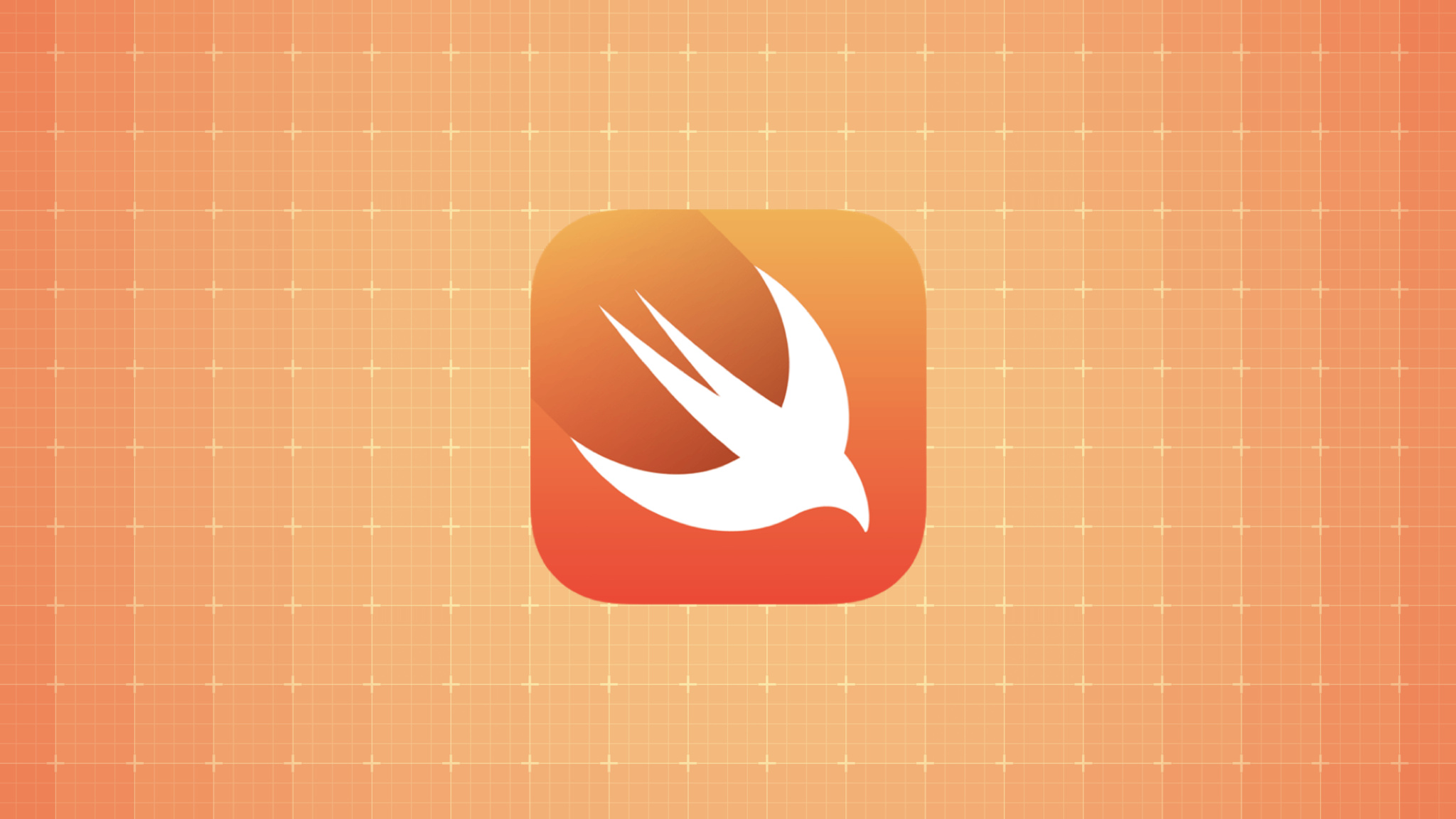
Observation Framework vs Combine vs @Published: Pick One
swift swiftui combine observation
Three ways to handle reactive state in SwiftUI: Combine, @Published, and Apple’s newer Observation framework. Apple loves adding choices. Developers hate making them. Let’s clear this mess up quickly.
🤷🏻 Combine: Powerful but Verbose
Combine was Apple’s first serious step into reactive programming. Powerful? Sure. Complex? Unfortunately.
import Combine
class ViewModel: ObservableObject {
@Published var name = ""
private var cancellables = Set<AnyCancellable>()
init() {
$name
.sink { newValue in
print("Name changed to \(newValue)")
}
.store(in: &cancellables)
}
}
Combine is precise and powerful. But pipelines, subscribers, publishers, cancellables? Verbose. Painful. Overkill for simple apps.
When Combine Wins:
- Complex pipelines (debouncing, throttling, merging streams)
- Precise control over subscriptions
😐 @Published: Simple but Limited
@Published + ObservableObject is minimal Combine. Just enough magic to feel nice:
class ViewModel: ObservableObject {
@Published var counter = 0
}
struct ContentView: View {
@StateObject var viewModel = ViewModel()
var body: some View {
VStack {
Text("\(viewModel.counter)")
Button("Increment") { viewModel.counter += 1 }
}
}
}
Easy to grasp. Easy to use. But limited. No transformations, no debouncing, no fine-grained subscription management.
When @Published Wins:
- Straightforward state updates
- Basic SwiftUI apps (90% of use-cases)
🚀 Observation Framework: Swift’s New Golden Child
New in Swift 5.9+, Observation is designed explicitly for SwiftUI’s simplicity:
@Observable class ViewModel {
var score = 0
}
struct ContentView: View {
@State var vm = ViewModel()
var body: some View {
VStack {
Text("Score: \(vm.score)")
Button("Increase") { vm.score += 1 }
}
}
}
It’s @Published done right. No boilerplate. No manual object lifecycle. No explicit ObservableObject. It’s lightweight and fits SwiftUI perfectly.
When Observation Wins:
- Lightweight, clean code
- Pure SwiftUI state management without boilerplate
- New SwiftUI-first projects (2024 and beyond)
💡 Recommendation: Use Observation (Most of the Time)
- Complex streams: Combine.
- Older or simple apps: @Published.
- Anything else? Observation.
Apple’s future lies clearly with Observation. Unless you’re maintaining legacy Combine-heavy codebases or building complex reactive flows, Observation is your new best friend.
Use it. Love it. Move forward.