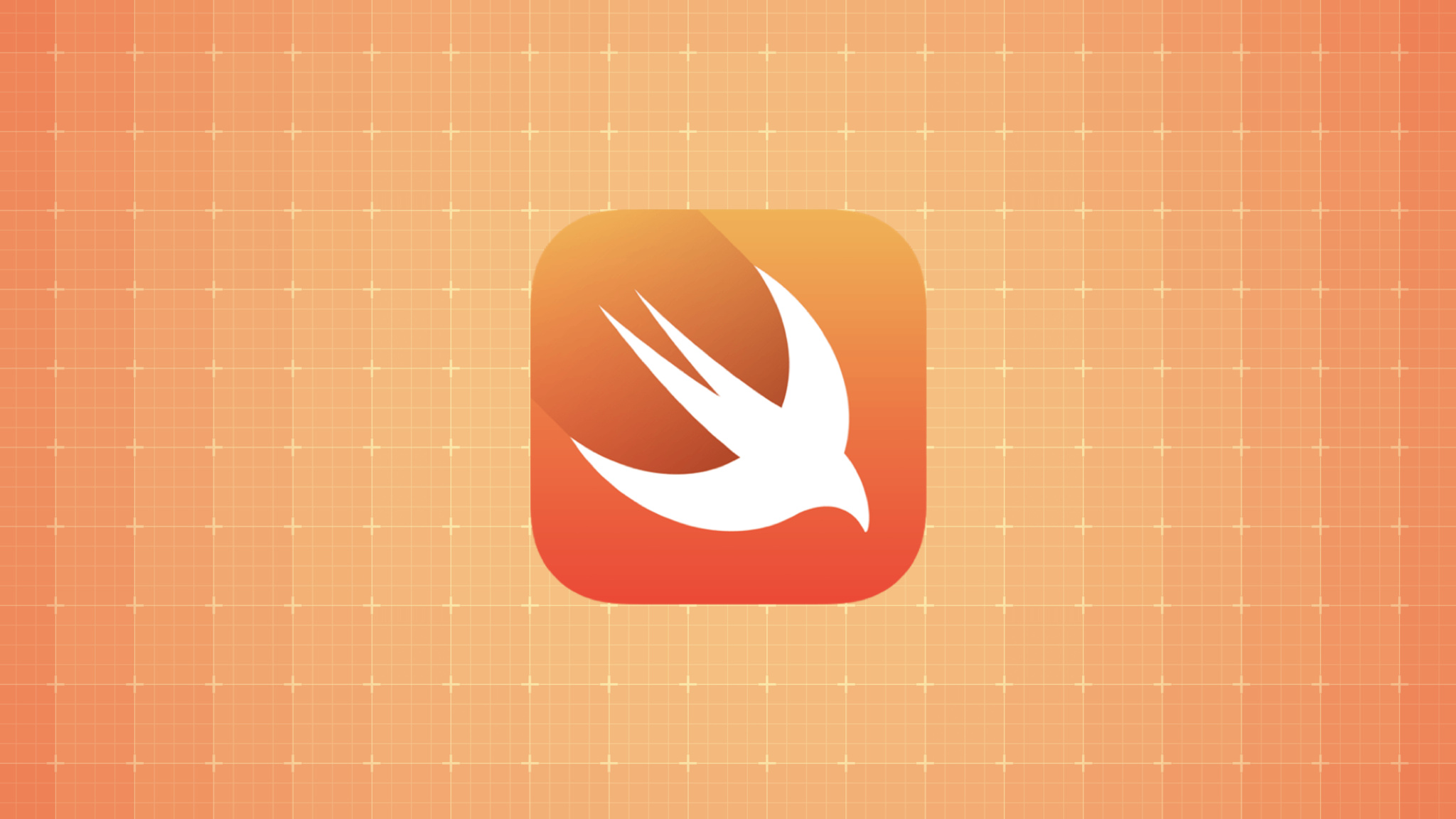
Swift Concurrency Two Years Later: Worth the Hype?
swift concurrency asyncawait
When Swift Concurrency dropped in late 2021, it promised to rewrite the rules. No more callback hell. No more racing dispatch queues. Just clean, readable async
/await
. Two years later, let’s ask: did it actually make things better?
Short answer: yes, but only if you use it right.
What Actually Works Well
🧵 Structured Concurrency Feels Like Real Engineering
Tasks live and die with their parent scope. No rogue threads. No zombie operations. Here’s how you can run tasks in parallel while still keeping your code elegant:
func loadUserProfile() async throws -> UserProfile {
async let userInfo = fetchUserInfo()
async let userPosts = fetchUserPosts()
return try await UserProfile(info: await userInfo, posts: await userPosts)
}
You kick off two async calls in parallel, then await them — structured and readable.
Pain Points That Still Hurt
😬 Cancellation Is There… Kinda
Tasks support cancellation. But if you don’t check Task.isCancelled manually, your async work keeps going anyway:
func loadImage(url: URL) async throws -> UIImage {
if Task.isCancelled { throw CancellationError() }
let (data, _) = try await URLSession.shared.data(from: url)
guard let image = UIImage(data: data) else {
throw ImageError.invalidData
}
return image
}
Forget to check cancellation? Your task wastes CPU and battery for no reason.
🪦 Detached Tasks Are a Trap
Detached tasks don’t inherit context — no structured cancellation, no actor isolation. Don’t abuse them.
Task {
await syncUserSettingsToCloud()
}
Instead, embed them in the right context:
@MainActor
func syncSettings() {
Task {
await syncUserSettingsToCloud()
}
}
Better yet, use TaskGroup or async let for scoped orchestration.
The Real MVP: Actors (But Also Kinda Overkill?)
Actors help isolate mutable state across threads. Here’s a basic example:
actor Counter {
private var value = 0
func increment() {
value += 1
}
func getValue() -> Int {
return value
}
}
But actor calls are async, and latency-prone. You also lose fine-grained control compared to something like a serial dispatch queue. Don’t use actors unless you need true thread isolation.
SwiftUI + Concurrency = 😍 (Mostly)
Concurrency fits naturally with SwiftUI’s task modifier:
struct ContentView: View {
@State private var user: User?
var body: some View {
VStack {
if let user = user {
Text("Welcome, \(user.name)")
} else {
ProgressView()
}
}
.task {
user = try? await fetchUser()
}
}
}
Clean. Declarative. Native. But it still needs guards, retries, and loading state management in real apps.
Final Thoughts
Swift Concurrency cleans up your code, adds guardrails, and encourages better architecture. It’s real progress. But it’s not magic. Cancellation, detached tasks, and actor performance can trip you up fast.
Two years later, it’s no longer hype — it’s table stakes. Use it wisely.