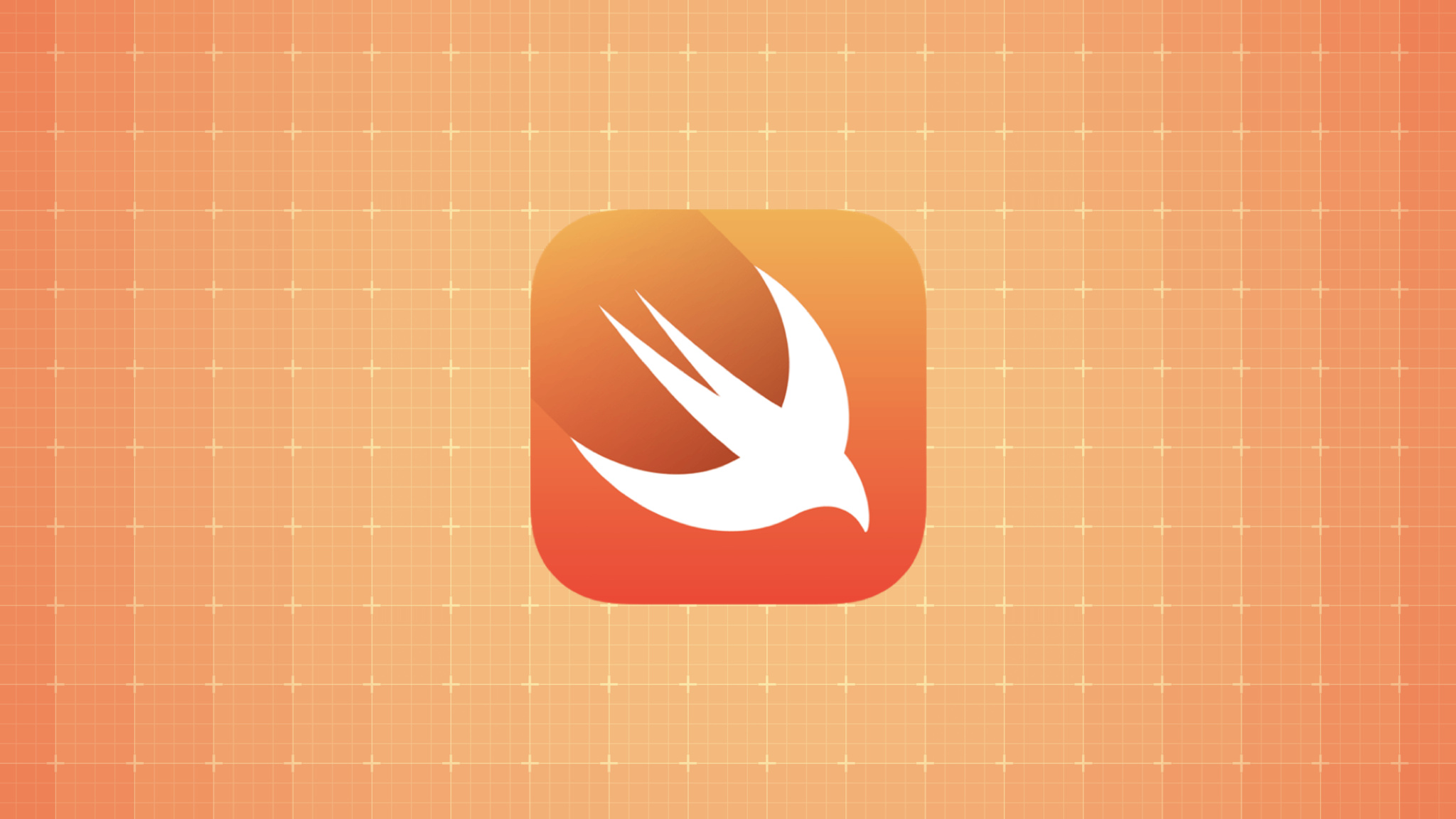
What's New in Swift 6: A Minimalist's Take
swift swift6 ios
Swift 6 is out. Let’s skip the marketing jargon and dive straight into the meaningful bits.
🧹 Simpler, Cleaner Type Inference
Less explicit typing, fewer headaches:
let numbers = [1, 2, 3, 4]
let evens = numbers.filter { $0.isMultiple(of: 2) } // Type inferred perfectly
Less clutter. More Swift.
🛡️ Noncopyable and Move-only Types
Control your memory explicitly:
moveonly struct Resource {
var handle: UnsafeRawPointer
}
func useResource(_ resource: consuming Resource) {
// resource consumed here, can't be reused later
}
Memory safety meets fine-grained control.
📦 Improved Macros & Compile-Time Evaluation
Macros now evaluate more efficiently at compile-time:
@AddAsync
func fetchUser() -> User { ... }
// Automatically generates async version at compile-time
Better macros = faster builds.
🎛️ Enhanced Concurrency: Task Groups and Isolation
Refined concurrency with clearer, safer code:
await withTaskGroup(of: Int.self) { group in
group.addTask { computeA() }
group.addTask { computeB() }
let sum = await group.reduce(0, +)
print(sum)
}
Concurrency without hidden complexity.
🎯 Pattern Matching Everywhere
More powerful and compact pattern matching:
if case let .success(value) = result {
print(value)
}
for case .admin(let user) in users {
print(user.name)
}
Concise, expressive Swift.
⚠️ Cleaner Error Handling
Simplified syntax for common patterns:
do {
try riskyCall()
} catch NetworkError.timeout {
retry()
} catch {
handleGenericError()
}
Readable. Simple. Explicit.
🧰 Standard Library Optimizations
Swift 6 stdlib is smaller, faster, and leaner—especially for apps running on constrained devices.
🤔 Should You Upgrade?
Yes. Swift 6 trims fat, improves safety, and enhances readability. It’s a meaningful step forward without disrupting your workflow.
Minimal fluff. Maximum benefit.